We had a database of hundreds of emails which were collected over years. The problem here is that some of the email exists, some do not. So while sending notifications to clients, the retuned invalid user mail messages require a lot of manual work to clear them. Our idea is to verify whether an email exists before sending the mail. Everything should be automatic and less time consuming.
The Solution
There were two solutions evolved explained below:
Above all, allow multi-threading to be used so that the delays can be shared.
How do the SMTP commands work?
It is worth learning how we can send emails manually using SMTP commands over telnet. For eg: Our interested email id is supercat@gmail.com. We can verify the email using the following steps:
Note: Our aim is to automate the commands through C#.
How does email verification with website work?
There are many online services which allows us to verify emails online. For eg:
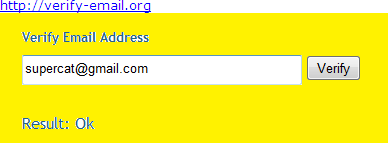
We can enter the email id and click the Verify button on website. If the email id exists it will return Result: Ok as reply.
Note: Our aim is to automate the form filling and submitting through C#. But the site only allows 20 emails to be verified per hour. So we need to find another site providing the service.
Implementation
As we analyzed both cases of verifying email, shall we jump into the implementation part? For implementing the MX Server lookup part we are using the following method:
public IList<MXServer> GetMXServers(string domainName)
{
string command = "nslookup -q=mx " + domainName;
ProcessStartInfo procStartInfo = new ProcessStartInfo("cmd", "/c " + command);
The Solution
There were two solutions evolved explained below:
- Automate nslookup and SMTP commands using C#
- Use WebClient automation to verify email using a website
Above all, allow multi-threading to be used so that the delays can be shared.
How do the SMTP commands work?
It is worth learning how we can send emails manually using SMTP commands over telnet. For eg: Our interested email id is supercat@gmail.com. We can verify the email using the following steps:
- Find the domain name from email. In the above case it is gmail.com
- Find the mail exchange server name from the domain name. Use command nslookup for achieving this: nslookup –q=mx gmail.com
The highlighted line shows that the gmail-smtp-in.l.google.com should be the high preference mail server. The actual preference should be treated as lower for a higher preference value.
- Connect to the mail server using port 25 and verify the email address. The commands would be:
telnet gmail-smtp-in.l.google.com 25
MAIL FROM: <sender@yourdomain.com>
RCPT TO: <supercat@gmail.com>
(If the email exists then the mail server will return 250 – if it doesn't then the value will be 550.)
Note: Our aim is to automate the commands through C#.
How does email verification with website work?
There are many online services which allows us to verify emails online. For eg:
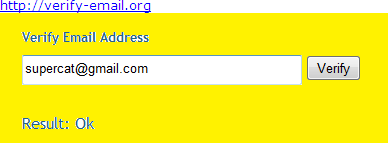
We can enter the email id and click the Verify button on website. If the email id exists it will return Result: Ok as reply.
Note: Our aim is to automate the form filling and submitting through C#. But the site only allows 20 emails to be verified per hour. So we need to find another site providing the service.
Implementation
As we analyzed both cases of verifying email, shall we jump into the implementation part? For implementing the MX Server lookup part we are using the following method:
public IList<MXServer> GetMXServers(string domainName)
{
string command = "nslookup -q=mx " + domainName;
ProcessStartInfo procStartInfo = new ProcessStartInfo("cmd", "/c " + command);
procStartInfo.RedirectStandardOutput = true;
procStartInfo.UseShellExecute = false;
procStartInfo.UseShellExecute = false;
procStartInfo.CreateNoWindow = true;
Process proc = new Process();
proc.StartInfo = procStartInfo;
proc.Start();
string result = proc.StandardOutput.ReadToEnd();
proc.StartInfo = procStartInfo;
proc.Start();
string result = proc.StandardOutput.ReadToEnd();
if (!string.IsNullOrEmpty(result))
result = result.Replace("\r\n", Environment.NewLine);
result = result.Replace("\r\n", Environment.NewLine);
IList<MXServer> list = new List<MXServer>();
foreach (string line in result.Split(new string[] { Environment.NewLine }, StringSplitOptions.RemoveEmptyEntries))
{
if (line.Contains("MX preference =") && line.Contains("mail exchanger ="))
{
MXServer mxServer = new MXServer();
mxServer.Preference = Int(GetStringBetween(line, "MX preference = ", ","));
mxServer.MailExchanger = GetStringFrom(line, "mail exchanger = ");
foreach (string line in result.Split(new string[] { Environment.NewLine }, StringSplitOptions.RemoveEmptyEntries))
{
if (line.Contains("MX preference =") && line.Contains("mail exchanger ="))
{
MXServer mxServer = new MXServer();
mxServer.Preference = Int(GetStringBetween(line, "MX preference = ", ","));
mxServer.MailExchanger = GetStringFrom(line, "mail exchanger = ");
list.Add(mxServer);
}
}
return list.OrderBy(m => m.Preference).ToList();
}
READ MORE>>
}
READ MORE>>
0 comments:
Post a Comment