Already loads of information are available on internet for the same purpose. But not all the code is placed at one place. So here is my small effort to accumulate all the code at one place and that is nothing better than this place. Hopefully it will be helpful for the beginners or whoever in needs of it.
Using the code
Namespace:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Net.Mail;
using System.Net;
using Outlook = Microsoft.Office.Interop.Outlook;
Block Diagram(SMTP Setting for various client):
Below is the Block diagram for the SMTP settings,have used to send out an email from various client providers.
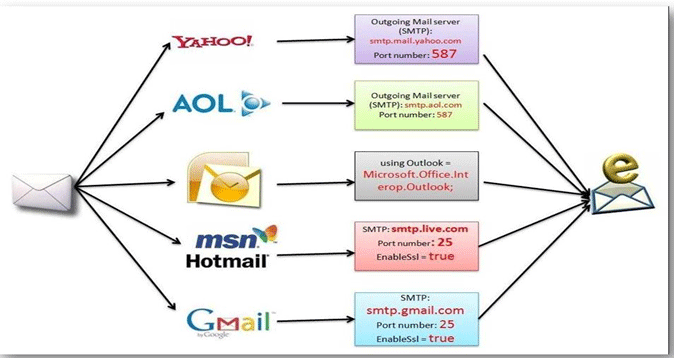
Wherever needed I have explain the code in details. The codes are self explanatory, any way for the same I have included comments. So here is the code snippet to achieve your purpose.
1. Using Outlook:
To send an email using outlook, we need to add a reference to the dynamic link library for Outlook which is called Microsoft.Office.Interop.Outlook.dll.
For the same follow the below steps
1. Go to your solution explorer
2. Click on add a reference
3. Click on .Net Tab
4. Go through the DLL and select Microsoft.Office.Interop.Outlook.dll correctly.
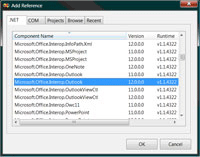
5. When you have selected the correct reference you select the "OK" button and this reference will be added to your project under references.
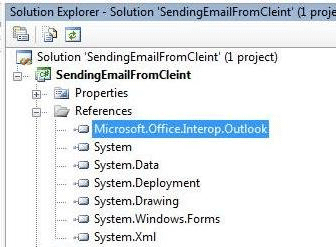
6. Now we need to add a reference in our class to the Outlook reference we have added to the project in our previous example.
using Outlook = Microsoft.Office.Interop.Outlook;
And finally the code would look something like this:
//method to send email to outlook
public void sendEMailThroughOUTLOOK()
{
try
{
// Create the Outlook application.
Outlook.Application oApp = new Outlook.Application();
// Create a new mail item.
Outlook.MailItem oMsg = (Outlook.MailItem)oApp.CreateItem(Outlook.OlItemType.olMailItem);
// Set HTMLBody.
//add the body of the email
oMsg.HTMLBody = "Hello, Jawed your message body will go here!!";
//Add an attachment.
String sDisplayName = "MyAttachment";
int iPosition = (int)oMsg.Body.Length + 1;
int iAttachType = (int)Outlook.OlAttachmentType.olByValue;
//now attached the file
Outlook.Attachment oAttach = oMsg.Attachments.Add(@"C:\\fileName.jpg", iAttachType, iPosition, sDisplayName);
//Subject line
oMsg.Subject = "Your Subject will go here.";
// Add a recipient.
Outlook.Recipients oRecips = (Outlook.Recipients)oMsg.Recipients;
// Change the recipient in the next line if necessary.
Outlook.Recipient oRecip = (Outlook.Recipient)oRecips.Add("jawed.ace@gmail.com");
oRecip.Resolve();
// Send.
oMsg.Send();
// Clean up.
oRecip = null;
oRecips = null;
oMsg = null;
oApp = null;
}//end of try block
catch (Exception ex)
{
}//end of catch
}//end of Email Method
2. Using HOTMAIL:
To send an email using HotMail, we need to add a reference to the dynamic link library for Hotmail/Gmail/AOL/Yahoo which is called System.Net.Mail.
For the same follow the below steps:
1. Go to solution explorer of your project
2. Select add a reference
3. Click on .Net Tab
4. Go through the DLL and select System.Net.Mail.
5. When you have selected the correct reference you select the "OK" button and this reference will be added to your project under references.
6. Now we need to add a reference in our class to the Hotmail/gmail/aol/yahoo reference we have added to the project.
using System.Net.Mail;
Note: The HOTMAIL SMTP Server requires an encrypted connection (SSL) on port 25.
And finally the code would look something like this:
//method to send email to HOTMAIL
public void sendEMailThroughHotMail()
{
try
{
//Mail Message
MailMessage mM = new MailMessage();
//Mail Address
mM.From = new MailAddress("sender@hotmail.com");
//receiver email id
mM.To.Add("rcver@gmail.com");
//subject of the email
mM.Subject = "your subject line will go here";
//deciding for the attachment
mM.Attachments.Add(new Attachment(@"C:\\attachedfile.jpg"));
//add the body of the email
mM.Body = "Body of the email";
mM.IsBodyHtml = true;
//SMTP client
SmtpClient sC = new SmtpClient("smtp.live.com");
//port number for Hot mail
sC.Port = 25;
//credentials to login in to hotmail account
sC.Credentials = new NetworkCredential("sender@hotmail.com","HotMailPassword");
//enabled SSL
sC.EnableSsl = true;
//Send an email
sC.Send(mM);
}//end of try block
catch (Exception ex)
{
}//end of catch
}
READ MORE>>
0 comments:
Post a Comment