How to easily send files (including Audio, Video, doc or any type of file) from Client to Server. System.Net.Sockets;using System.IO;
Then write code for the button1 (Browse) and the button2 (Send) as in the following.
For example:
CLIENT PROGRAM:
using System;
It is necessary to specify the server's "Computer Name" in the TcpClient space like:
TcpClient client = new TcpClient("SwtRascal", 5055);
In this the TcpClient specified the Computer Name as "SwtRascal".
First we have to design a form for the client such as:
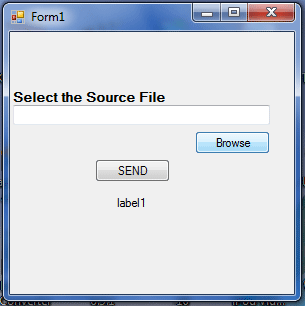
And place an OpenFileDialog from the ToolBox-> Dialogs-> OpenFileDialog control.
Then Double-Click the form; the coding page will open; in that specify the following namespaces:
using
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;using System.Drawing;
using System.Text;using System.Windows.Forms;
using System.Net.Sockets;
using System.IO;
namespace filee
{
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
string n;
byte[] b1;
OpenFileDialog op;
Then write code for the button1 (Browse) and the button2 (Send) as in the following.
For example:
CLIENT PROGRAM:
using System;
It is necessary to specify the server's "Computer Name" in the TcpClient space like:
TcpClient client = new TcpClient("SwtRascal", 5055);
In this the TcpClient specified the Computer Name as "SwtRascal".
First we have to design a form for the client such as:
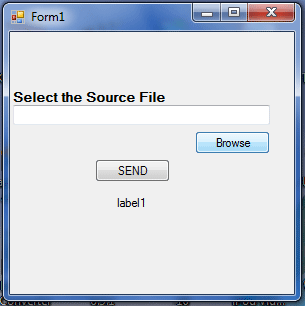
And place an OpenFileDialog from the ToolBox-> Dialogs-> OpenFileDialog control.
Then Double-Click the form; the coding page will open; in that specify the following namespaces:
using
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;using System.Drawing;
using System.Text;using System.Windows.Forms;
using System.Net.Sockets;
using System.IO;
namespace filee
{
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
string n;
byte[] b1;
OpenFileDialog op;
private void button1_Click(object sender, EventArgs e)
{
op = new OpenFileDialog();
if (op.ShowDialog() == DialogResult.OK)
{
string t = textBox1.Text;
t = op.FileName;
FileInfo fi = new FileInfo(textBox1.Text = op.FileName);
n = fi.Name + "." + fi.Length;
TcpClient client = new TcpClient("SwtRascal", 5055);
StreamWriter sw = new StreamWriter(client.GetStream());
sw.WriteLine(n);
sw.Flush();
label1.Text = "File Transferred....";
}
{
op = new OpenFileDialog();
if (op.ShowDialog() == DialogResult.OK)
{
string t = textBox1.Text;
t = op.FileName;
FileInfo fi = new FileInfo(textBox1.Text = op.FileName);
n = fi.Name + "." + fi.Length;
TcpClient client = new TcpClient("SwtRascal", 5055);
StreamWriter sw = new StreamWriter(client.GetStream());
sw.WriteLine(n);
sw.Flush();
label1.Text = "File Transferred....";
}
}
private void button2_Click(object sender, EventArgs e)
{
TcpClient client = new TcpClient("SwtRascal", 5050);
Stream s = client.GetStream();
b1 = File.ReadAllBytes(op.FileName);
s.Write(b1, 0, b1.Length);
client.Close();
label1.Text = "File Transferred....";
{
TcpClient client = new TcpClient("SwtRascal", 5050);
Stream s = client.GetStream();
b1 = File.ReadAllBytes(op.FileName);
s.Write(b1, 0, b1.Length);
client.Close();
label1.Text = "File Transferred....";
}
}}
For Server : Open a new C# Windows Application form.
Create and design a Form for Server like:
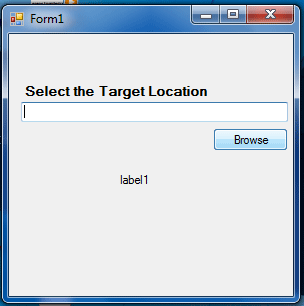
Then Place a folderBrowserDialog from the ToolBox->Dialogs-> folderBrowserDialog.
After Designing the Form, Double-Click the form; the coding page will open; in that specify the following namespaces:
using System.Net.Sockets;using System.IO;using System.Net;
Then write code for the button1 (Browse) and for the Form Load function, such as in the following.
If you want to specipy an IP Address for the system then include code such as:
Instead of using the code TcpListener list = new TcpListener(port1);
It only specifies the port address.
Use this code.
It specifies the IP Address and Port.
IPAddress localAddr = IPAddress.Parse("192.168.1.20"); TcpListener list = new TcpListener(localAddr, port);
Below the Comment line shows the ipaddress option..
For example:
SERVER PROGRAM:
using System;using System.Collections.Generic;using System.ComponentModel;using System.Data;using System.Drawing;using System.Text;using System.Windows.Forms;using System.Net.Sockets;using System.IO;using System.Net;
}}
For Server : Open a new C# Windows Application form.
Create and design a Form for Server like:
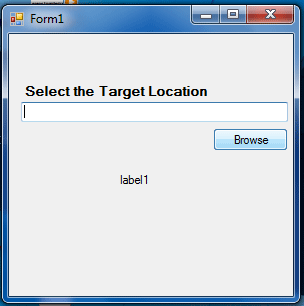
Then Place a folderBrowserDialog from the ToolBox->Dialogs-> folderBrowserDialog.
After Designing the Form, Double-Click the form; the coding page will open; in that specify the following namespaces:
using System.Net.Sockets;using System.IO;using System.Net;
Then write code for the button1 (Browse) and for the Form Load function, such as in the following.
If you want to specipy an IP Address for the system then include code such as:
Instead of using the code TcpListener list = new TcpListener(port1);
It only specifies the port address.
Use this code.
It specifies the IP Address and Port.
IPAddress localAddr = IPAddress.Parse("192.168.1.20"); TcpListener list = new TcpListener(localAddr, port);
Below the Comment line shows the ipaddress option..
For example:
SERVER PROGRAM:
using System;using System.Collections.Generic;using System.ComponentModel;using System.Data;using System.Drawing;using System.Text;using System.Windows.Forms;using System.Net.Sockets;using System.IO;using System.Net;
namespace filee
{
public partial class Form2 : Form {
public Form2()
{
InitializeComponent();
}
string rd;
byte[] b1;
string v;
int m;
TcpListener list;
{
public partial class Form2 : Form {
public Form2()
{
InitializeComponent();
}
string rd;
byte[] b1;
string v;
int m;
TcpListener list;
Int32 port = 5050;
Int32 port1 = 5055;
//IPAddress localAddr = IPAddress.Parse("192.168.1.20");
Int32 port1 = 5055;
//IPAddress localAddr = IPAddress.Parse("192.168.1.20");
private void Browse_Click(object sender, EventArgs e)
{
{
if (folderBrowserDialog1.ShowDialog() == DialogResult.OK)
{
textBox1.Text = folderBrowserDialog1.SelectedPath;
//TcpListener list = new TcpListener(localAddr,port1); list = new TcpListener(port1);
list.Start();
TcpClient client = list.AcceptTcpClient();
Stream s = client.GetStream();
b1 = new byte[m];
s.Read(b1, 0, b1.Length);
File.WriteAllBytes(textBox1.Text + "\\" + rd.Substring(0, rd.LastIndexOf('.')), b1);
list.Stop();
client.Close();
label1.Text = "File Received......";
}
{
textBox1.Text = folderBrowserDialog1.SelectedPath;
//TcpListener list = new TcpListener(localAddr,port1); list = new TcpListener(port1);
list.Start();
TcpClient client = list.AcceptTcpClient();
Stream s = client.GetStream();
b1 = new byte[m];
s.Read(b1, 0, b1.Length);
File.WriteAllBytes(textBox1.Text + "\\" + rd.Substring(0, rd.LastIndexOf('.')), b1);
list.Stop();
client.Close();
label1.Text = "File Received......";
}
}
private void Form2_Load(object sender, EventArgs e)
{
//TcpListener list = new TcpListener(localAddr, port); TcpListener list = new TcpListener(port);
list.Start();
TcpClient client = list.AcceptTcpClient();
MessageBox.Show("Client trying to connect");
StreamReader sr = new StreamReader(client.GetStream());
rd = sr.ReadLine();
v = rd.Substring(rd.LastIndexOf('.') + 1);
m = int.Parse(v);
list.Stop();
client.Close();
{
//TcpListener list = new TcpListener(localAddr, port); TcpListener list = new TcpListener(port);
list.Start();
TcpClient client = list.AcceptTcpClient();
MessageBox.Show("Client trying to connect");
StreamReader sr = new StreamReader(client.GetStream());
rd = sr.ReadLine();
v = rd.Substring(rd.LastIndexOf('.') + 1);
m = int.Parse(v);
list.Stop();
client.Close();
}
}}
After Designing the Forms for Client and Server, run the Server first and after that run the Client.
READ MORE>>
After Designing the Forms for Client and Server, run the Server first and after that run the Client.
READ MORE>>
0 comments:
Post a Comment